This article is a bit different from my usual column in two ways. First, it's starting with a hardware and software combo—something I've not done before. Second, the projects are linked to each other and come recommended to me by Perth LUG member, Simon Newton.
Given the mostly hardware-based information for the project, here are some carefully selected bits of information from the Web site:
Arduino is an open-source electronics prototyping platform based on flexible, easy-to-use hardware and software. It's intended for artists, designers, hobbyists and anyone interested in creating interactive objects or environments.
Arduino can sense the environment by receiving input from a variety of sensors and can affect its surroundings by controlling lights, motors and other actuators. The microcontroller on the board is programmed using the Arduino programming language and the Arduino development environment. Arduino projects can be standalone or they can communicate with software running on a computer.
The open-source Arduino environment makes it easy to write code and upload it to the I/O board. It runs on Windows, Mac OS X and Linux. The environment is written in Java and based on Processing, avr-gcc and other open source-software.
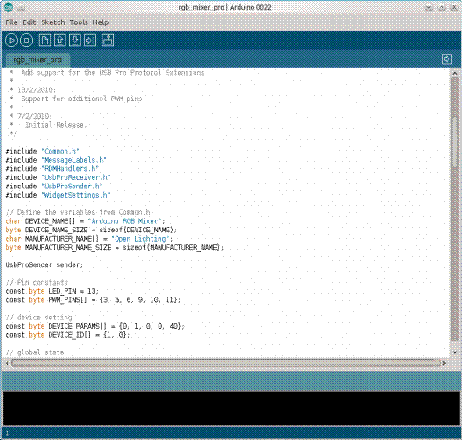
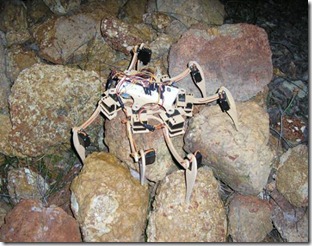
Installation
For those who are happy with a binary, the Web site makes things very easy with 32- and 64-bit binary tarballs at the download page, and if you're lucky, the Arduino IDE may even be in your repository. If you're going with the binary tarball, just download the latest from the Web site, extract it, and open a terminal in the folder. To run the program, enter the command:
If you're running from source instead, instructions are available on the Web site with a link from the Downloads page, although I don't have the space here to cover its somewhat unusual installer method. Nevertheless, it does recommend a series of packages that should help troubleshoot mishaps with both the source and binary tarballs. The Web site says you need the following: Sun Java SDK, avr-gcc, avr-g++, avr-libc, make, ant and git.
If you have a local repository version installed, chances are the program can be started with this command:
$ arduino
Under my Kubuntu installation, the Arduino IDE was available in the KDE menu under Applications→Electronics→Arduino IDE.
However, I must stop you here before actually running the program, and I apologize if I led you astray in the last few paragraphs (don't worry if you've already started it, you can close and re-open it with no worries). Obviously, before you can do anything with an Arduino board and the software, you first have to plug in your Arduino device. This will help in the configuration of your hardware, especially if you're using a USB connection.
Once that's out of the way, you now can start the program with any of the methods above.
Usage
With the program running and the device plugged in, let's set it up. Inside the main window, click on the Tools menu and navigate your way to the Board menu. From there, choose your Arduino device (I had the Arduino Uno). Now you have to choose your serial port, which is under Tools→Serial Port. If you had a USB device and the program found it, a USB option should appear here (in my case, /dev/ttyUSB0).
With all of that boring stuff out of the way, I'm sure you're keen to sink your teeth into this hardware/software combo. The IDE makes things simple with a series of examples in an easy-access menu. Look under File→Examples, and check out the impressive list of examples from which to choose. I recommend starting with Blink under the 1.Basics menu.
With Blink, you can start with the most basic of basics and come to grasp the syntax with well laid out code with documentation for each line. To try out this code, click Upload, which is the sixth button along in the blue menu, with the right-facing arrow. If all goes well, you should see your device start blinking from an LED, perhaps with a board reset in the process.
If your board has an enabled reset facility like the Uno I was using, you should be able to make code changes by uploading them, watching the board next to you reset and start again with the new program. In fact, I recommend you try it now. Change one of the lines, perhaps one of the lines dealing with the delay time, and then upload it again. Now this may seem lame, but to a hardware "n00b" like myself, changing around the program and updating the running hardware in a visible way was quite a buzz!
If you want to check out your code before uploading it, the start and stop buttons are for verifying the code, with the stop button obviously allowing you to cancel any compiling partway through. Although I'm running out of space for the software side, I recommend checking out more of the examples in the code, where genuinely real-world uses are available. Some highlights include ChatServer, "a simple server that distributes any incoming messages to all connected clients"; a reader for barometric pressure sensors; and a program for demonstrating and controlling sprite animations.
However, I've been neglecting one of Arduino's real bonuses, and that is the ability to use a board to program any number of chips, remove them from the main Arduino board, and use them to run external devices. The nature of open hardware really makes this a robotic enthusiast's wet dream, with examples like my close mate Phil's robotic spider showing some of the cool things you can achieve with this suite.
Nevertheless, I do have one specific use of Arduino in mind to tie this column together, and that is Simon Newton's Arduino RGB Mixer: a six channel color mixer that interfaces with OLA. Check out the following link for instructions on how to make this simple device that shows off both of these projects at the same time:
http://www.opendmx.net/index.php/Arduino_RGB_Mixer.

Simon Newton's RGB Mixer is a great way to use both Arduino and OLA together.Read more at: http://arduino.cc/en
Getting Started – The Basics
This example contains the bare minimum of code you need for an Arduino sketch to compile: the setup() method and the loop()method.
Hardware Required
Arduino Board
Circuit
Only your Arduino Board is needed for this example.

image developed using Fritzing. For more circuit examples, see the Fritzing project page
Code
The setup() function is called when a sketch starts. Use it to initialize variables, pin modes, start using libraries, etc. The setup function will only run once, after each powerup or reset of the Arduino board.
After creating a setup() function, the loop() function does precisely what its name suggests, and loops consecutively, allowing your program to change and respond as it runs. Code in the loop() section of your sketch is used to actively control the Arduino board.
The code below won't actually do anything, but it's structure is useful for copying and pasting to get you started on any sketch of your own. It also shows you how to make comments in your code.
Any line that starts with two slashes (//) will not be read by the compiler, so you can write anything you want after it. Commenting your code like this can be particularly helpful in explaining, both to yourself and others, how your program functions step by step.
void setup() {
// put your setup code here, to run once:
}
void loop() {
// put your main code here, to run repeatedly:
}
Getting Started – Hello World (Blink)
This example shows the simplest thing you can do with an Arduino to see physical output: it blinks an LED.
Hardware Required
· Arduino Board
· LED
Circuit
To build the circuit, attach a 220-ohm resistor to pin 13. Then attach the long leg of an LED (the positive leg, called the anode) to the resistor. Attach the short leg (the negative leg, called the cathode) to ground. Then plug your Arduino board into your computer, start the Arduino program, and enter the code below.
Most Arduino boards already have an LED attached to pin 13 on the board itself. If you run this example with no hardware attached, you should see that LED blink.
click the image to enlarge
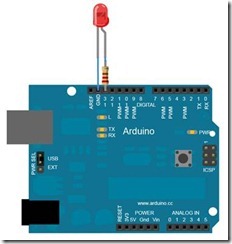
image developed using Fritzing. For more circuit examples, see the Fritzing project page
Schematic
click the image to enlarge
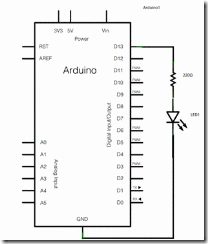
Code
In the program below, the first thing you do is to initialize pin 13 as an output pin with the line
pinMode(13, OUTPUT);
In the main loop, you turn the LED on with the line:
digitalWrite(13, HIGH);
This supplies 5 volts to pin 13. That creates a voltage difference across the pins of the LED, and lights it up. Then you turn it off with the line:
digitalWrite(13, LOW);
That takes pin 13 back to 0 volts, and turns the LED off. In between the on and the off, you want enough time for a person to see the change, so the delay() commands tell the Arduino to do nothing for 1000 milliseconds, or one second. When you use the delay() command, nothing else happens for that amount of time. Once you've understood the basic examples, check out the BlinkWithoutDelay example to learn how to create a delay while doing other things.
Once you've understood this example, check out the DigitalReadSerial example to learn how read a switch connected to the Arduino.
/*
Blink
Turns on an LED on for one second, then off for one second, repeatedly.
This example code is in the public domain.
*/
void setup() {
// initialize the digital pin as an output.
// Pin 13 has an LED connected on most Arduino boards:
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH); // set the LED on
delay(1000); // wait for a second
digitalWrite(13, LOW); // set the LED off
delay(1000); // wait for a second
}
See Also
- DigitalReadSerial: Read a switch, print the state out to the Arduino Serial Monitor.
- AnalogReadSerial: Read a potentiometer, print it's state out to the Arduino Serial Monitor.
- Fade: Demonstrates the use of analog output to fade an LED.
Based On